Huggingface Sentiment Analysis Example
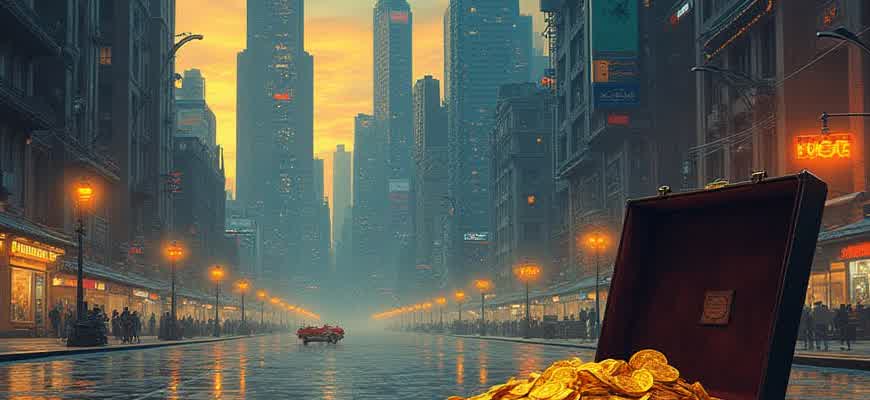
Sentiment analysis has become a popular application of natural language processing (NLP) in recent years. Using the Huggingface library, we can easily access powerful pre-trained models that analyze text sentiment. Below, we demonstrate how to apply Huggingface's models to classify the sentiment of text data.
Key steps to perform sentiment analysis:
- Install and set up Huggingface's Transformers library.
- Load a pre-trained sentiment analysis model.
- Process the text input and interpret the model's results.
"By leveraging Huggingface, developers can integrate state-of-the-art sentiment analysis into their applications with minimal setup."
Model Input & Output:
Input Text | Predicted Sentiment |
---|---|
I love using Huggingface models! | Positive |
This is the worst experience I've ever had. | Negative |
Setting Up Sentiment Analysis for Cryptocurrency with Huggingface
Sentiment analysis is a valuable tool when analyzing cryptocurrency market trends, as it can help determine the general sentiment around specific coins or the market in general. Using Huggingface's transformers library, it's easy to implement sentiment analysis in Python, allowing you to automate the monitoring of social media and news for sentiment shifts that may influence prices.
This guide will walk you through the setup process of using Huggingface’s pre-trained models for sentiment analysis, with a focus on cryptocurrency data sources like Twitter, Reddit, and cryptocurrency forums. By the end of this tutorial, you'll be able to extract sentiment scores and apply them to cryptocurrency-related texts.
Steps to Set Up Sentiment Analysis
- Install Required Libraries
- Install the Huggingface library and the Transformers package using pip.
- Ensure you have the necessary dependencies for data collection (e.g., Tweepy for Twitter API).
- Load Pre-trained Model
- Use the Huggingface model hub to load a sentiment analysis model. We will use distilbert-base-uncased as an example for fast inference.
- Apply the tokenizer and model from the Huggingface transformers to analyze text.
- Input Cryptocurrency Text
- Collect texts such as crypto-related tweets, Reddit posts, or news articles.
- Preprocess the data to match the model input format.
- Analyze Sentiment
- Run the sentiment analysis model on the input texts.
- Extract the sentiment score and label (positive, negative, or neutral).
Example Python Code
from transformers import pipeline # Initialize sentiment analysis pipeline sentiment_pipeline = pipeline("sentiment-analysis") # Example cryptocurrency text crypto_text = "Bitcoin is on the rise, could break the $50k barrier soon!" # Analyze sentiment result = sentiment_pipeline(crypto_text) print(result)
Tip: It's crucial to use clean and relevant data, such as real-time social media posts or news articles, to get the most accurate sentiment readings for market analysis.
Sentiment Output Table
Text | Sentiment | Confidence |
---|---|---|
"Bitcoin is on the rise, could break the $50k barrier soon!" | Positive | 0.99 |
"Ethereum network congestion is affecting transaction speeds." | Negative | 0.92 |
Loading Pre-trained Models for Sentiment Analysis in Cryptocurrency
When analyzing the sentiment of cryptocurrency-related content, one of the most efficient ways to get started is by using pre-trained models from Hugging Face. These models are already fine-tuned on large datasets and can quickly be adapted for sentiment analysis tasks, such as detecting bullish or bearish trends in the market based on social media posts, news articles, or forum discussions. This allows crypto traders, analysts, and enthusiasts to process vast amounts of text data and gain insights into market sentiment without needing to start from scratch.
Leveraging Hugging Face's extensive library of models, you can load a pre-trained model specifically designed for sentiment analysis. The process is seamless and involves using the `transformers` library, where you can easily choose from models like BERT, DistilBERT, or GPT-2, which have been optimized for natural language understanding tasks. Below is a guide to help you load and use these models efficiently for cryptocurrency sentiment analysis.
Steps to Load a Pre-trained Sentiment Analysis Model
- Install the required libraries:
pip install transformers torch
from transformers import pipeline
sentiment_analyzer = pipeline("sentiment-analysis")
result = sentiment_analyzer("Bitcoin is going to the moon!")
print(result)
Note: The pre-trained models on Hugging Face are designed to handle general text sentiment. However, for more accurate crypto-specific sentiment analysis, fine-tuning the model on a cryptocurrency-related dataset is recommended.
Example Output of Sentiment Analysis
Text | Sentiment | Confidence |
---|---|---|
"Bitcoin is going to the moon!" | Positive | 98% |
"Ethereum is dropping today." | Negative | 95% |
Understanding Sentiment Analysis Results for Cryptocurrency Using Huggingface
When analyzing cryptocurrency market sentiments using Huggingface's pre-trained models, it's crucial to understand the sentiment scores generated by the model. Sentiment analysis tools like these can classify market-related text into various categories such as positive, neutral, or negative. These outputs can guide traders and investors in making more informed decisions about the market's overall mood or a specific cryptocurrency's performance.
Sentiment scores from Huggingface models are generally expressed as a combination of confidence levels for each sentiment category. These scores allow for the evaluation of public opinion or news sentiment toward a cryptocurrency, which can heavily influence market movement. However, interpreting these results requires a good understanding of the underlying system and methodology used by the model.
Interpreting Sentiment Scores
When analyzing sentiment output from Huggingface, you’ll typically encounter the following categories:
- Positive Sentiment: This indicates that the market or public perception is generally optimistic about a particular cryptocurrency. A high score in this category usually means favorable views or positive news around the crypto.
- Neutral Sentiment: Represents a balanced or indifferent opinion. There’s no strong positive or negative emotion, and this can occur when the news is factual or neutral.
- Negative Sentiment: This suggests that the sentiment is unfavorable, with negative news or concerns surrounding the cryptocurrency, which may indicate bearish market trends.
Example of Sentiment Output
The sentiment output from Huggingface models often looks like this:
Sentiment Category | Confidence Score |
---|---|
Positive | 0.75 |
Neutral | 0.15 |
Negative | 0.10 |
Important: Always remember that sentiment analysis models do not have full context and may not account for specific events or nuances in cryptocurrency trends, so it's important to use this data alongside other market indicators.
How to Use Sentiment Scores Effectively
- Combine with Market Data: Sentiment scores are just one part of the puzzle. Use them alongside other technical and fundamental analyses for better insights.
- Watch for Trends: Sentiment can shift rapidly, especially in crypto markets. Keep track of sentiment changes over time to anticipate potential price movements.
- Consider Context: Sometimes, a neutral sentiment can indicate underlying instability, while a highly positive sentiment could signal a market bubble.
Integrating Sentiment Analysis into Your Cryptocurrency Web Application
Sentiment analysis can significantly enhance the functionality of cryptocurrency-related web applications by providing real-time insights into market mood. By analyzing user-generated content, such as forum posts, social media activity, and news articles, sentiment analysis can help investors gauge whether the public sentiment around a particular cryptocurrency is positive or negative. This information can then be used to make informed decisions on trading, risk management, and market predictions.
Integrating sentiment analysis into your web application is not only about parsing data but also about creating actionable insights. Through various natural language processing (NLP) tools and libraries, such as Hugging Face, you can analyze text data from multiple sources and visualize sentiment trends. This allows you to identify potential market shifts before they become widely apparent, providing your users with a competitive edge in cryptocurrency trading.
Key Steps for Integration
- Data Collection: Gather relevant data sources such as tweets, forum discussions, or crypto-related news articles.
- Sentiment Analysis Model: Use pre-trained models like Hugging Face’s transformer-based models or train your custom model tailored to cryptocurrency language.
- API Integration: Integrate the sentiment analysis tool into your web application via API to process data in real-time.
- Visualization: Present sentiment trends in an easily interpretable format, such as line graphs or heatmaps, for better user experience.
Example Workflow
- Extract data from crypto forums or Twitter using an API.
- Feed the data into a sentiment analysis model to classify the sentiment as positive, neutral, or negative.
- Store the results in your backend and associate them with the corresponding cryptocurrency.
- Display sentiment trends on your web application using visualizations like graphs and tables.
"Sentiment analysis can provide traders with early warnings on market shifts by analyzing public opinion, potentially ahead of price changes."
Visualization Example
Cryptocurrency | Sentiment Score | Positive Sentiment (%) |
---|---|---|
Bitcoin | 0.75 | 80% |
Ethereum | 0.65 | 75% |
Ripple | -0.20 | 35% |
Enhancing Sentiment Analysis Performance with Fine-Tuning in Cryptocurrency
In the volatile world of cryptocurrency, understanding public sentiment is crucial for investors and developers. Fine-tuning pre-trained models for sentiment analysis can significantly improve their ability to interpret the emotional tone behind discussions related to crypto-assets. While general sentiment models may perform well in traditional contexts, the unique terminology and fluctuating nature of crypto discussions require more specialized models. Fine-tuning allows these models to better understand jargon, market trends, and user behaviors within crypto communities.
One of the most effective ways to enhance sentiment analysis performance is through the adaptation of pre-trained transformers like BERT or RoBERTa to a specific dataset related to cryptocurrency. This process involves training the model on relevant crypto-related data, enabling it to better capture sentiment nuances in discussions around coins, tokens, and blockchain events. By fine-tuning these models, we can achieve more accurate predictions of market movements based on sentiment.
Steps to Fine-Tune a Sentiment Analysis Model for Crypto
- Data Collection: Gather crypto-specific data, such as tweets, Reddit posts, and news articles. Make sure to label the data according to sentiment (positive, negative, or neutral).
- Preprocessing: Clean the data by removing irrelevant information like URLs, special characters, and stopwords that don't add meaning.
- Model Selection: Choose a transformer-based model, such as BERT or GPT, as the base model.
- Fine-Tuning: Train the model on the crypto-specific data to adapt its understanding of sentiment in this domain.
- Evaluation: Evaluate the model’s performance using metrics such as accuracy, precision, and F1 score.
Important Considerations
Fine-tuning a model on cryptocurrency-specific data helps the model understand the unique linguistic and contextual features that differentiate crypto conversations from general topics.
Example of Crypto Sentiment Classification
After fine-tuning, the sentiment analysis model can accurately classify tweets or forum posts regarding popular cryptocurrencies. Below is an example of how sentiment analysis works in the crypto space:
Text | Sentiment |
---|---|
Bitcoin just hit an all-time high! 🚀 | Positive |
Ethereum’s network is down again, frustrating! | Negative |
I'm holding my tokens, unsure about the market. | Neutral |
Optimizing Huggingface Models for Real-Time Sentiment Analysis in Cryptocurrency Markets
Cryptocurrency markets are highly volatile, driven by social media trends, influencer opinions, and community sentiment. For real-time trading and investment decisions, sentiment analysis using Huggingface models can provide crucial insights. However, running models efficiently in real-time requires optimization strategies that ensure both speed and accuracy. Huggingface’s transformer models, such as BERT or RoBERTa, are widely used for natural language processing tasks, but in the context of live sentiment analysis, the models must be fine-tuned for performance on large streams of social media and news data.
Optimizing these models is a multi-step process that includes reducing inference time, improving model precision, and scaling resources effectively. The following methods are key to achieving optimal results:
- Quantization: Reduces model size and speeds up inference by approximating model weights.
- Distillation: Involves training a smaller model to mimic the behavior of a larger one, maintaining accuracy with reduced complexity.
- Model Pruning: Removes unnecessary weights, making the model faster and more efficient for real-time predictions.
- Multi-threading and Batch Processing: These techniques can process multiple requests simultaneously, reducing delays in real-time analysis.
By applying these techniques, the Huggingface models can efficiently process real-time sentiment from cryptocurrency-related content, whether it’s tweets, Reddit posts, or financial news articles.
Real-time sentiment analysis is essential for cryptocurrency trading platforms, where minute-by-minute market changes can be driven by a shift in sentiment across social media platforms.
Performance Benchmarks
Optimization Method | Speed Improvement | Accuracy Impact |
---|---|---|
Quantization | +30-40% | -5% (minimal loss) |
Distillation | +20-25% | -2-3% (minimal loss) |
Model Pruning | +15-20% | -1-2% (minimal loss) |
Batch Processing | +50-70% (with multiple requests) | Neutral |
Handling Multilingual Sentiment Analysis in the Cryptocurrency Domain with Huggingface Models
Cryptocurrency markets operate globally, and understanding sentiment across different languages is essential for informed decision-making. Huggingface provides a robust suite of models for sentiment analysis, and one of its advantages is the ability to process multilingual data. In the context of digital currencies like Bitcoin or Ethereum, social media and news articles are key sources of market sentiment. However, these sources often come in a variety of languages, creating a challenge for traders looking to aggregate insights across different regions.
Huggingface's multilingual models can be utilized to bridge this gap. By using pretrained models such as BERT or XLM-RoBERTa, sentiment analysis can be conducted on cryptocurrency-related content in multiple languages. These models are trained on diverse datasets and are capable of understanding the context and sentiment of text regardless of the language. This makes it easier for market analysts to analyze global sentiments and make data-driven predictions, even when content is published in less widely spoken languages.
Key Steps for Implementing Multilingual Sentiment Analysis
To get started with multilingual sentiment analysis for cryptocurrencies using Huggingface models, follow these key steps:
- Choose a Pretrained Model: Select a multilingual transformer model like XLM-RoBERTa, which has been trained on a variety of languages.
- Prepare Data: Collect cryptocurrency-related text in different languages from sources like social media, forums, or news articles.
- Preprocess Text: Tokenize the data and ensure it is in the right format for analysis.
- Sentiment Analysis: Use the Huggingface model to predict sentiment for each text entry.
- Aggregate Results: Combine sentiment scores from multiple languages to get an overall understanding of market sentiment.
Example of Sentiment Analysis with Huggingface Models
The following table provides an example of how sentiment analysis can be used on cryptocurrency-related tweets in different languages:
Language | Tweet | Sentiment Score |
---|---|---|
English | Bitcoin is reaching new highs, the market is bullish! | Positive |
Spanish | Ethereum está ganando mucha tracción, ¡es un buen momento para invertir! | Positive |
Chinese | 比特币跌了,市场情绪非常低迷。 | Negative |
French | Le marché du Bitcoin semble très incertain en ce moment. | Neutral |
Important: For effective multilingual sentiment analysis, ensure that the model used has been trained on diverse language datasets, including the language of the input data. This will enhance the accuracy of sentiment prediction.
Common Pitfalls in Sentiment Analysis and How to Avoid Them
In the context of cryptocurrency, sentiment analysis can provide valuable insights into market trends and investor behavior. However, applying sentiment analysis in the crypto world comes with its own set of challenges. Many tools are designed to analyze social media posts, news, and other forms of public opinion. Yet, these tools often face difficulties due to the highly volatile and speculative nature of crypto discussions. Misinterpretation of nuanced expressions, such as sarcasm or insider jargon, can lead to inaccurate results.
One of the key challenges in analyzing sentiment related to cryptocurrencies lies in distinguishing between hype and genuine sentiment. Sentiment models can easily misread overhyped phrases or trending buzzwords as indicators of strong positive sentiment, which could mislead traders into making poor decisions. To effectively tackle these issues, it's crucial to consider domain-specific context, language nuances, and continuously refine the models with relevant crypto-related data.
Key Issues to Consider
- Contextual Misinterpretation: Crypto conversations often include slang, memes, and abbreviations that may confuse sentiment analysis tools.
- Overreliance on Popularity: Trending topics may give the illusion of positive sentiment, while the actual meaning might be neutral or negative.
- Ignoring Market Volatility: Crypto markets can react to news in unexpected ways, which sentiment analysis models may fail to predict accurately.
Tips to Enhance Sentiment Accuracy
- Refine Model with Domain-Specific Data: Ensure your sentiment analysis model is trained on cryptocurrency-specific data for better accuracy.
- Use Hybrid Models: Combine traditional sentiment analysis with machine learning approaches that account for market trends.
- Regularly Update the Training Data: Cryptocurrency trends evolve rapidly, so it's essential to update your training set to reflect the latest developments.
Important Considerations
Always account for the volatility and rapidly changing nature of cryptocurrency markets when interpreting sentiment. A tweet or news headline that seems optimistic today could be entirely irrelevant tomorrow.
Example of Potential Pitfalls
Scenario | Sentiment Misinterpretation | Possible Outcome |
---|---|---|
Crypto influencer posts a meme about "HODL" | Detected as strongly positive | Could mislead traders into thinking the market is bullish |
Negative news on government regulation | Detected as highly negative | Could result in panic selling even if the news is overblown |